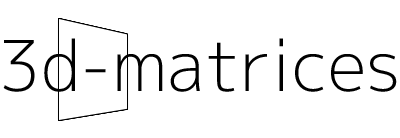
3d matrices
1.0.0A utility library implementing 2x2, 3x3, 4x4, and NxN matrix functionality.
About 3d-matrices
This is a library implementing common matrix operations, mainly intended as the counterpiece to 3d-vectors and thus being aimed at operations in 3D space. Still, it also implements other common matrix tasks such as LU and QR factorisation, determinant computation, and sub-matrix selection. 2x2, 3x3, and 4x4 matrices are specially treated and often have specifically optimised or inlined variants in the operations to ensure as high a speed as possible. NxM matrices are also available, but will always use a general algorithm in the operations.
This library by no means attempts, nor comes in any way close to replacing or imitating things such as BLAS and LIN/LAPACK. The main purpose is to have a library that allows convenient matrix operations in conjunction with the 3d-vectors library. It should be sufficiently fast and accurate for most purposes, but should not be used for serious matrix based calculations. Please use industry-standard packages for that.
How To
Load it through ASDF or Quicklisp and use the package.
(ql:quickload :3d-matrices)
(use-package :3d-matrices)
All the functions are prefixed with an m
or with nm
for destructive ops. This should ensure that there are no clashes in names. Now let's look at creating matrices.
(mat 1 2 3 4)
(mat2 1)
(mat2 '(1 2 3 4))
(mcopy (mat3))
(matn 2 3)
(meye 5)
(muniform 2 3 1)
(mrand 10 10)
In order to see the matrix in a more human-readable format, you can use describe
or write-matrix
directly:
(describe (meye 5))
(write-matrix (mat2) T)
(write-matrix (mat2) T :format :wolfram)
(write-matrix (mat2) T :format :array)
Matrices always use float
s. Where sensible, operations will accept real
s as well however. Either single-float
s or double-float
s are used, depending on the presence of the :3d-vectors-double-floats
keyword in *features*
. This feature is taken over from the 3d-vectors library to ensure that both of them always agree on the float type.
The type mat
includes all subtypes mat2
, mat3
, mat4
, and matn
. Each of them have their own specific accessors that are suffixed with the dimension number. Usually you should be fine with using the generic variants, but if you already know the type you should probably fall back to using the specific one, or use with-fast-matref
.
(miref (meye 2) 3)
(mcref (meye 2) 1 1)
(with-fast-matref (e (mat 2) 2)
(e 1 1))
Matrices are basically a struct that contains a simple-vector of floats. This means that every single reference must also dereference the array first. This is why, if you have many repeated accesses to an array, you should use with-fast-matref
or do the same manually by first retrieving the backing array with marr
.
If you're coming to this library with the intention of using it to do 3D math, you'll most likely be mostly looking for how to create translation, rotation, and scaling matrices. Specific functions exist for this that take care of it for you. They all operate on mat4
s and take a vec3
as argument.
(let ((mat (mtranslation (vec 1 2 3))))
(nmscale mat (vec 1 2 3))
(nmrotate mat +vx+ 90)
(m* mat (vec 1 2 3 4)))
Aside from translations you'll probably also want to set up a projection and a camera. You can do this, too.
(mperspective 75 (/ w h) 0.001 10000) ; Perspective projection
(mortho 0 w h 0 0.001 10000) ; Orthographic projection
(nmlookat modelview camera-pos center +vy+) ; Look at the centre
Aside from the basic comparison operators m=
m~=
m/=
m<
m>
m<=
m>=
, and arithmetic operators m+
m-
m*
m/
nm+
nm-
nm*
n*m
nm/
, 3d-matrices also includes LU decomposition mlu
, determinant computation mdet
, inversion minv
, transposition mtranspose
, trace calculation mtrace
, minors mminor
, cofactors mcof
mcofactor
, matrix adjugate madj
, pivoting mpivot
, norming m1norm
minorm
m2norm
, QR decomposition mqr
and eigenvalue calculation meigen
. These should all work "as you expect" and I will thus refrain from showing them off in detail here. Refer to your standard linear algebra textbook to get an understanding of what they do if you don't know already.
Finally, There's also some basic operators to do sectioning or restructuring of a matrix.
(mcol (mat 1 2 3 4) 0)
(mrow (mat 1 2 3 4) 0)
(mdiag (mat 1 0 0 0 2 0 0 0 3))
(mtop (mat 1 2 3 4 5 6 7 8 9) 2)
(nmswap-row (mat 1 2 3 4) 0 1)
And that's pretty much all she wrote. Note that some operations will only work on square or non-singular matrices, and all operations that take multiple operands require them to be of a compatible type. For example, you can only multiply matrices that are of agreeable rows and columns or multiply with a vector that is of the appropriate size.
Also See
System Information
Definition Index
-
3D-MATRICES
- ORG.SHIRAKUMO.FLARE.MATRIX
No documentation provided.-
EXTERNAL STRUCTURE MAT2
The 2x2 matrix type.
-
EXTERNAL STRUCTURE MAT3
The 3x3 matrix type.
-
EXTERNAL STRUCTURE MAT4
The 4x4 matrix type.
-
EXTERNAL STRUCTURE MATN
The NxM matrix type.
-
EXTERNAL TYPE-DEFINITION MAT
-
EXTERNAL FUNCTION M*
- VAL
- &REST
- VALS
Computes a matrix multiplication. If the other operand is a real, the matrix is multiplied with the real element-wise. If the other operand is a matrix, they are multiplied as per matrix multiplication. Note that the returned matrix may have different size than the input matrices as a result of this. The two matrices must agree on the size as per matrix multiplication.
-
EXTERNAL FUNCTION M+
- VAL
- &REST
- VALS
Computes the element-wise addition of the matrices or reals. Returns a fresh matrix.
-
EXTERNAL FUNCTION M-
- VAL
- &REST
- VALS
Computes the element-wise subtraction of the matrices or reals. Returns a fresh matrix.
-
EXTERNAL FUNCTION M/
- VAL
- &REST
- VALS
Computes an element-wise division of the matrix from a real. Returns a fresh matrix.
-
EXTERNAL FUNCTION M/=
- VAL
- &REST
- VALS
Compares matrices against each other or a real, returning T if they are element-wise equal by /=.
-
EXTERNAL FUNCTION M1NORM
- M
Computes the 1 norm of the matrix, namely the maximum of the sums of the columns.
-
EXTERNAL FUNCTION M2NORM
- M
Computes the 2 norm of the matrix, namely the square root of the sum of all squared elements.
-
EXTERNAL FUNCTION M<
- VAL
- &REST
- VALS
Compares matrices against each other or a real, returning T if they are element-wise ordered by <.
-
EXTERNAL FUNCTION M<=
- VAL
- &REST
- VALS
Compares matrices against each other or a real, returning T if they are element-wise ordered by <=.
-
EXTERNAL FUNCTION M=
- VAL
- &REST
- VALS
Compares matrices against each other or a real, returning T if they are element-wise equal by =.
-
EXTERNAL FUNCTION M>
- VAL
- &REST
- VALS
Compares matrices against each other or a real, returning T if they are element-wise ordered by >.
-
EXTERNAL FUNCTION M>=
- VAL
- &REST
- VALS
Compares matrices against each other or a real, returning T if they are element-wise ordered by >=.
-
EXTERNAL FUNCTION MADJ
- M
Computes the adjugate of the matrix. For MAT2 MAT3 MAT4, inlined variants exist. For MATN, an algorithm based on the cofactors is used.
-
EXTERNAL FUNCTION MAPPLY
- MAT
- OP
Applies the function to each element of the matrix and maps the result of it to a new matrix.
-
EXTERNAL FUNCTION MAPPLYF
- MAT
- OP
Applies the function to each element of the matrix and maps the result of it back into the matrix.
-
EXTERNAL FUNCTION MARR
- MAT
-
EXTERNAL FUNCTION MARR2
- INSTANCE
Direct accessor to the backing array of the MAT2.
-
EXTERNAL FUNCTION (SETF MARR2)
- VALUE
- INSTANCE
No documentation provided. -
EXTERNAL FUNCTION MARR3
- INSTANCE
Direct accessor to the backing array of the MAT3o.
-
EXTERNAL FUNCTION (SETF MARR3)
- VALUE
- INSTANCE
No documentation provided. -
EXTERNAL FUNCTION MARR4
- INSTANCE
Direct accessor to the backing array of the MAT4.
-
EXTERNAL FUNCTION (SETF MARR4)
- VALUE
- INSTANCE
No documentation provided. -
EXTERNAL FUNCTION MARRN
- INSTANCE
Direct accessor to the backing array of the MATN.
-
EXTERNAL FUNCTION (SETF MARRN)
- VALUE
- INSTANCE
No documentation provided. -
EXTERNAL FUNCTION MAT
- &REST
- VALS
-
EXTERNAL FUNCTION MAT-P
- MAT
-
EXTERNAL FUNCTION MAT2
- &OPTIONAL
- ELEMENTS
Constructs a MAT2 from the given elements. ELEMENTS can be NULL --- The matrix is initialised with zeroes. REAL --- The matrix is initialised with this number. SEQUENCE --- The sequence is mapped into the matrix and the rest are initialised to 0.
-
EXTERNAL FUNCTION MAT2-P
- OBJECT
Returns T if the given object is of type MAT2.
-
EXTERNAL FUNCTION MAT3
- &OPTIONAL
- ELEMENTS
Constructs a MAT3 from the given elements. ELEMENTS can be NULL --- The matrix is initialised with zeroes. REAL --- The matrix is initialised with this number. SEQUENCE --- The sequence is mapped into the matrix and the rest are initialised to 0.
-
EXTERNAL FUNCTION MAT3-P
- OBJECT
Returns T if the given object is of type MAT3.
-
EXTERNAL FUNCTION MAT4
- &OPTIONAL
- ELEMENTS
Constructs a MAT4 from the given elements. ELEMENTS can be NULL --- The matrix is initialised with zeroes. REAL --- The matrix is initialised with this number. SEQUENCE --- The sequence is mapped into the matrix and the rest are initialised to 0.
-
EXTERNAL FUNCTION MAT4-P
- OBJECT
Returns T if the given object is of type MAT4.
-
EXTERNAL FUNCTION MATF
- MAT
- &REST
- VALS
Maps the VALs into the matrix. The values will be mapped in row-major order.
-
EXTERNAL FUNCTION MATN
- R
- C
- &OPTIONAL
- ELEMENTS
Constructs a MATN of the requested size from the given elements. ELEMENTS can be NULL --- The matrix is initialised with zeroes. REAL --- The matrix is initialised with this number. SEQUENCE --- The sequence is mapped into the matrix and the rest are initialised to 0. Note that if R and C are both... 2 - A MAT2 is constructed 3 - A MAT3 is constructed 4 - A MAT4 is constructed instead of a MATN.
-
EXTERNAL FUNCTION MATN-P
- OBJECT
Returns T if the given object is of type MATN.
-
EXTERNAL FUNCTION MBLOCK
- M
- Y1
- X1
- Y2
- X2
Returns the designated sub-matrix as a new matrix. Y1, X1 are the upper left corner, inclusive Y2, X2 are the lower right corner, exclusive
-
EXTERNAL FUNCTION MBOTTOM
- M
- N
Returns the lowermost N rows as a new matrix.
-
EXTERNAL FUNCTION MCOF
- M
Computes the cofactor matrix. See MCOFACTOR
-
EXTERNAL FUNCTION MCOFACTOR
- M
- Y
- X
Computes the cofactor at the specified index of the matrix. This is just the element at the position multiplied by the minor. See MMINOR
-
EXTERNAL FUNCTION MCOL
- MAT
- N
Accesses the requested column as a vector of the appropriate size. This only works for MAT2, MAT3, MAT4.
-
EXTERNAL FUNCTION (SETF MCOL)
- VEC
- MAT
- N
No documentation provided. -
EXTERNAL FUNCTION MCOLS
- MAT
Returns the number of columns the matrix stores.
-
EXTERNAL FUNCTION MCOPY
- M
-
EXTERNAL FUNCTION MCOPY2
- M2
Creates a full copy of the MAT2.
-
EXTERNAL FUNCTION MCOPY3
- M3
Creates a full copy of the MAT3.
-
EXTERNAL FUNCTION MCOPY4
- M4
Creates a full copy of the MAT4.
-
EXTERNAL FUNCTION MCOPYN
- MN
Creates a full copy of the MATN.
-
EXTERNAL FUNCTION MCREF
- MAT
- Y
- X
-
EXTERNAL FUNCTION MCREF2
- MAT
- Y
- X
Returns the element at the given cell in the MAT2.
-
EXTERNAL FUNCTION MCREF3
- MAT
- Y
- X
Returns the element at the given cell in the MAT3.
-
EXTERNAL FUNCTION MCREF4
- MAT
- Y
- X
Returns the element at the given cell in the MAT4.
-
EXTERNAL FUNCTION MCREFN
- MAT
- Y
- X
Returns the element at the given cell in the MATN.
-
EXTERNAL FUNCTION MDET
- M
Computes the determinant of the matrix. For MAT2 MAT3 MAT4, inlined variants exist. For MATN, an algorithm based on LU factorisation is used.
-
EXTERNAL FUNCTION MDIAG
- M
Returns the diagonal values of the matrix as a list.
-
EXTERNAL FUNCTION MEIGEN
- M
- &OPTIONAL
- ITERATIONS
Computes an approximation of the eigenvalues of the matrix. An approach based on QR factorisation is used. The number of iterations dictates how many times the factorisation is repeated to make the result more accurate. Usually something around 50 iterations should give somewhat accurate results, but due to floating point limitations that may be off more significantly. Returns the eigenvalues as a list.
-
EXTERNAL FUNCTION MEYE
- N
Constructs a square identity matrix of the requested size.
-
EXTERNAL FUNCTION MFRUSTUM
- LEFT
- RIGHT
- BOTTOM
- TOP
- NEAR
- FAR
Returns a 3D frustum projection view matrix. See MPERSPECTIVE
-
EXTERNAL FUNCTION MINORM
- M
Computes the infinity norm of the matrix, namely the maximum of the sums of the rows.
-
EXTERNAL FUNCTION MINV
- M
Computes the inverses of the matrix. This is only possible if the determinant is non-zero. For MAT2 MAT3 MAT4, inlined variants exist. For MATN, an algorithm based on the adjugate is used.
-
EXTERNAL FUNCTION MIREF
- MAT
- I
-
EXTERNAL FUNCTION MIREF2
- MAT
- I
Returns the element at the given index in the MAT2. Elements are stored in row-major format.
-
EXTERNAL FUNCTION MIREF3
- MAT
- I
Returns the element at the given index in the MAT3. Elements are stored in row-major format.
-
EXTERNAL FUNCTION MIREF4
- MAT
- I
Returns the element at the given index in the MAT4. Elements are stored in row-major format.
-
EXTERNAL FUNCTION MIREFN
- MAT
- I
Returns the element at the given index in the MATN. Elements are stored in row-major format.
-
EXTERNAL FUNCTION MLEFT
- M
- N
Returns the leftmost N columns as a new matrix.
-
EXTERNAL FUNCTION MLOOKAT
- EYE
- TARGET
- UP
Returns a view translation matrix that should "look at" TARGET from EYE where UP is the up vector.
-
EXTERNAL FUNCTION MLU
- M
- &OPTIONAL
- PIVOT
Computes an LU factorisation of the matrix. An approach based on Crout is used with on-the-fly pivotisation if requested. Returns the combined LU matrix, the permutation matrix, and the number of permutations that were done.
-
EXTERNAL FUNCTION MMINOR
- M
- Y
- X
Computes the minor at the specified index of the matrix. This basically calculates the determinant of the matrix with the row and column of the specified index excluded.
-
EXTERNAL FUNCTION MORTHO
- LEFT
- RIGHT
- BOTTOM
- TOP
- NEAR
- FAR
Returns a 3D orthographic projection view matrix.
-
EXTERNAL FUNCTION MPERSPECTIVE
- FOVY
- ASPECT
- NEAR
- FAR
Returns a 3D perspective projection view matrix. FOVY -- The field of view (how "zoomy" it is) ASPECT -- The aspect ratio of the screen NEAR / FAR -- The Z near and far clipping planes See MFRUSTUM
-
EXTERNAL FUNCTION MPIVOT
- M
Attempts to do a partial pivotisation. Returns the pivotised matrix, the permutation matrix, and the number of permutations that were done.
-
EXTERNAL FUNCTION MQR
- MAT
Computes the QR factorisation of the matrix. An approach based on givens rotations is used. Returns the Q and R matrices, which are fresh.
-
EXTERNAL FUNCTION MRAND
- R
- C
- &KEY
- MIN
- MAX
Constructs a matrix of the requested size where each element is randomized. MIN and MAX return the inclusive bounds of the numbers in the matrix.
-
EXTERNAL FUNCTION MRIGHT
- M
- N
Returns the rightmost N columns as a new matrix.
-
EXTERNAL FUNCTION MROTATION
- V
- ANGLE
Returns a 3D rotation matrix for the given vector as a MAT4.
-
EXTERNAL FUNCTION MROW
- MAT
- N
Accesses the requested row as a vector of the appropriate size. This only works for MAT2, MAT3, MAT4.
-
EXTERNAL FUNCTION (SETF MROW)
- VEC
- MAT
- N
No documentation provided. -
EXTERNAL FUNCTION MROWS
- MAT
Returns the number of rows the matrix stores.
-
EXTERNAL FUNCTION MSCALING
- V
Returns a 3D scaling matrix for the given vector as a MAT4.
-
EXTERNAL FUNCTION MTOP
- M
- N
Returns the topmost N rows as a new matrix.
-
EXTERNAL FUNCTION MTRACE
- M
Computes the trace of the matrix. For MAT2 MAT3 MAT4, inlined variants exist. For MATN, a generic sum is used.
-
EXTERNAL FUNCTION MTRANSLATION
- V
Returns a 3D translation matrix for the given vector as a MAT4.
-
EXTERNAL FUNCTION MTRANSPOSE
- M
Computes the transpose of the matrix. For MAT2 MAT3 MAT4, inlined variants exist. For MATN, a generic swap is used.
-
EXTERNAL FUNCTION MUNIFORM
- R
- C
- ELEMENT
Constructs a matrix of the requested size where each element is initialised to the requested element.
-
EXTERNAL FUNCTION M~=
- VAL
- &REST
- VALS
Compares matrices against each other or a real, returning T if they are element-wise equal by ~=. See ~=
-
EXTERNAL FUNCTION N*M
- VAL
- &REST
- VALS
Computes a modifying matrix multiplication, but modifying the right-hand side. See NM*
-
EXTERNAL FUNCTION NM*
- VAL
- &REST
- VALS
Computes a modifying matrix multiplication. If the other operand is a real, the matrix is multiplied with the real element-wise. If the other operand is a matrix, they are multiplied as per matrix multiplication. Note that this only works for square matrix against square matrix, as otherwise a size change would occur, which is not possible to do in a modifying variant. The two matrices must agree on the size as per matrix multiplication. If the other operand is a vector, the vector is modified. See N*M
-
EXTERNAL FUNCTION NM+
- VAL
- &REST
- VALS
Computes the element-wise addition of the matrices or reals. Returns the first matrix, modified.
-
EXTERNAL FUNCTION NM-
- VAL
- &REST
- VALS
Computes the element-wise subtraction of the matrices or reals. Returns the first matrix, modified.
-
EXTERNAL FUNCTION NM/
- VAL
- &REST
- VALS
Computes an element-wise division of the matrix from a real. Returns the modified, first matrix.
-
EXTERNAL FUNCTION NMLOOKAT
- M
- EYE
- TARGET
- UP
Modifies the matrix to look at TARGET from the EYE. See MLOOKAT.
-
EXTERNAL FUNCTION NMROTATE
- M
- V
- ANGLE
Rotates the given matrix around the vector by angle. Returns the modified matrix.
-
EXTERNAL FUNCTION NMSCALE
- M
- V
Scales the given matrix by the vector. Returns the modified matrix.
-
EXTERNAL FUNCTION NMSWAP-COL
- M
- K
- L
Modifies the matrix by swapping the Kth column with the Lth column.
-
EXTERNAL FUNCTION NMSWAP-ROW
- M
- K
- L
Modifies the matrix by swapping the Kth row with the Lth row.
-
EXTERNAL FUNCTION NMTRANSLATE
- M
- V
Translates the given matrix by the vector. Returns the modified matrix.
-
EXTERNAL FUNCTION WRITE-MATRIX
- M
- STREAM
- &KEY
- FORMAT
Writes the matrix in a certain format, by default a human-readable one. FORMAT can be one of :NICE - Prints it in a nice representation intended for humans. :WOLFRAM - Prints it in the format for Wolfram Alpha, namely {{a,b..},..} :ARRAY - Prints it as a common lisp 2D array. If the STREAM is NIL, a string of the output is returned. Otherwise the matrix itself is returned.
-
EXTERNAL MACRO WITH-FAST-MATCASE
- ACCESSOR
- MAT
- &BODY
- BODY
Does an etypecase on MAT and an appropriate WITH-FAST-MATREF on each case. The body should be an expression of (MAT-TYPE form*). For each matrix type /except/ for MATN, the forms will be wrapped in an appropriate WITH-FAST-MATREF. This is not done for MATN, as often times a different approach to manipulating the matrix than by direct reference is preferable for that case. See WITH-FAST-MATREF
-
EXTERNAL MACRO WITH-FAST-MATREF
- ACCESSOR
- MAT
- WIDTH
- &BODY
- BODY
Allows efficient referencing to matrix elements. ACCESSOR designates the name of the local macro that will allow you to both read and set the matrix element at the given position It will take either one or two arguments. If two, they are row and column of the cell to dereference, and if one, it is the row-major index of the element to dereference. You must also designate the proper number of columns stored in the matrix. This is done because often times when you will want to use this macro, you'll already know the number of columns anyway. Retrieving it again would be wasteful. You should use this whenever you need to reference elements in a loop or need to do more than one reference overall.
-
EXTERNAL MACRO WITH-FAST-MATREFS
- BINDINGS
- &BODY
- BODY
Allows efficient referencing of multiple matrices. Each binding must be of the form that WITH-FAST-MATREF expects. See WITH-FAST-MATREF